Vector graphics
Vector graphics are a form of computer graphics in which visual images are created directly from geometric shapes defined on a Cartesian plane, such as points, lines, curves and polygons.
The associated mechanisms may include vector display and printing hardware, vector data models and file formats, as well as the software based on these data models (especially graphic design software, computer-aided design, and geographic information systems).
Vector graphics is an alternative to raster or bitmap graphics, with each having advantages and disadvantages in specific situations.
Vector file types:
-
.ai
: Short for Adobe Illustrator, this file is commonly used in print media and digital graphics, such as logos. -
.eps
: Encapsulated PostScript is an older type of vector graphics file. .eps files don’t support transparency in the way more modern file formats like .ai do. -
.pdf
: The Portable Document Format is built for the exchange of documents across platforms and is editable in Adobe Acrobat. -
.svg
: The Scalable Vector Graphics format is based in XML (a mark-up language used widely across the Internet that’s readable by both machines and humans). It’s useful for the web, where it can be indexed, searched and scripted.
SVG
Scalable Vector Graphics (SVG) is an XML-based vector image format for defining two-dimensional graphics, having support for interactivity and animation.
- An SVG file is a standard graphics file type used for rendering images on the internet.
- SVG files work best for images that contain less detail than a photograph.
- All modern browsers support rendering, interpreting, and displaying SVG files. To open a
.svg
file, launch your browser, then open the file and it will show in your browser.
Basic example
The following code will create a image:
<svg xmlns="http://www.w3.org/2000/svg" viewBox="0 0 24 24" style="width:1rem;height:1rem;fill:currentColor">
<g data-name="Layer 2"><g data-name="external-link">
<rect width="24" height="24" opacity="0"></rect>
<path d="M20 11a1 1 0 0 0-1 1v6a1 1 0 0 1-1 1H6a1 1 0 0 1-1-1V6a1 1 0 0 1 1-1h6a1 1 0 0 0 0-2H6a3 3 0 0 0-3 3v12a3 3 0 0 0 3 3h12a3 3 0 0 0 3-3v-6a1 1 0 0 0-1-1z"></path>
<path d="M16 5h1.58l-6.29 6.28a1 1 0 0 0 0 1.42 1 1 0 0 0 1.42 0L19 6.42V8a1 1 0 0 0 1 1 1 1 0 0 0 1-1V4a1 1 0 0 0-1-1h-4a1 1 0 0 0 0 2z"></path>
</g></g>
</svg>
When put this code in HTML file, it will display a image:
SVG Features
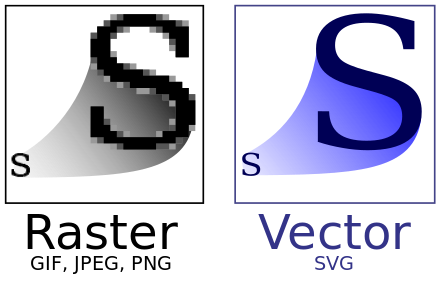
- SVG supports interactivity, animation, and rich graphical capabilities, making it suitable for both web and print applications.
- SVG allows three types of graphic objects: vector graphic shapes (such as paths consisting of straight lines and curves), bitmap images, and text.
- SVG also supports metadata, enabling better indexing, searching, and retrieval of SVG content.
- Though the SVG Specification primarily focuses on vector graphics markup language, its design includes the basic capabilities of a page description language like Adobe’s PDF.
- Despite its benefits, SVG can pose security risks if used for images, as it can host scripts or CSS, potentially leading to cross-site scripting attacks or other vulnerabilities.
SVG Can Be
-
Icons. Most icons can be translated well to vectors, given their simplicity and clearly defined borders. Icons for page elements like buttons will need to be responsive for varying screen sizes, which means they must be perfectly scalable.
-
Logos. The SVG format is particularly well suited for logos, which appear in website headers, emails, and in print on anything from pamphlets to hoodies to billboards. Again, logos tend to be simpler in design, which lends nicely to the SVG format.
-
Illustrations. Vectors also suit non-photo visual art nicely. Decorative drawings on webpages can both scale easily and conserve file space if added as SVG files. You can create the illustrations below, even the textures on some shapes, with SVGs.
-
Animations and Interface Elements. By harnessing the capabilities of CSS and JavaScript, you can set SVGs to change their appearance dynamically and to trigger automatically during or after an event. Animated SVGs can serve to add visual flair to your pages, or you can use them to engage with user interface animations.
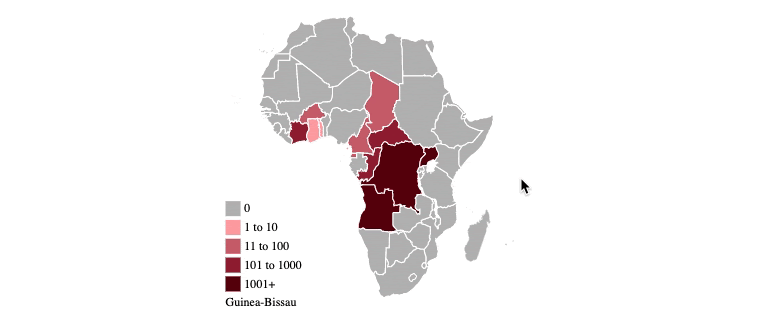
SVG Animation
Animation of SVG is possible through various means:
- Scripting: ECMAScript is a primary means of creating animations and interactive user interfaces within SVG.
- Styling: Since 2008, the development of CSS Animations as a feature in WebKit has made possible stylesheet-driven implicit animation of SVG files from within the Document Object Model (DOM).
- SMIL: (Synchronized Multimedia Integration Language), a recommended means of animating SVG-based hypermedia
Using SMIL
<!DOCTYPE svg PUBLIC "-//W3C//DTD SVG 1.1//EN" "http://www.w3.org/Graphics/SVG/1.1/DTD/svg11.dtd">
<svg version="1.1" xmlns="http://www.w3.org/2000/svg" xmlns:xlink="http://www.w3.org/1999/xlink"
width="100%" height="100%" viewBox="-4 -4 8 8">
<title>SVG animation using SMIL</title>
<circle cx="0" cy="1" r="2" stroke="red" fill="none">
<animateTransform
attributeName="transform"
attributeType="XML"
type="rotate"
from="0"
to="360"
begin="0s"
dur="1s"
repeatCount="indefinite"/>
</circle>
</svg>
Other SMIL sample (Morphing_SMIL.svg):
Using CSS Animation
<!DOCTYPE svg PUBLIC "-//W3C//DTD SVG 1.1//EN" "http://www.w3.org/Graphics/SVG/1.1/DTD/svg11.dtd">
<svg version="1.1" xmlns="http://www.w3.org/2000/svg" xmlns:xlink="http://www.w3.org/1999/xlink"
width="100%" height="100%" viewBox="-4 -4 8 8">
<title>SVG animation using CSS</title>
<style type="text/css">
@keyframes rot_kf {
from { transform: rotate(0deg); }
to { transform: rotate(360deg); }
}
.rot { animation: rot_kf 1s linear infinite; }
</style>
<circle class="rot"
cx="0" cy="1" r="2" stroke="blue" fill="none"/>
</svg>
Using ECMAScript
<!DOCTYPE svg PUBLIC "-//W3C//DTD SVG 1.1//EN" "http://www.w3.org/Graphics/SVG/1.1/DTD/svg11.dtd">
<svg version="1.1" xmlns="http://www.w3.org/2000/svg" xmlns:xlink="http://www.w3.org/1999/xlink" width="100%" height="100%" viewBox="-4 -4 8 8" onload="rotate(evt)">
<title>SVG animation using ECMAScript</title>
<script type="text/ecmascript">
function rotate(evt) {
var object = evt.target.ownerDocument.getElementById('rot');
setInterval(function () {
var now = new Date();
var milliseconds = now.getTime() % 1000;
var degrees = milliseconds * 0.36; // 360 degrees in 1000 ms
object.setAttribute('transform', 'rotate(' + degrees + ')');
}, 20);
}
</script>
<circle id="rot"
cx="0" cy="1" r="2" stroke="green" fill="none"/>
</svg>
Disadvantages of SVG
-
Not the right format for high-quality or detailed photos. Designers create vector graphics with points and paths, not pixels. So, you can create a vector graphic by tracing a photograph or using a converter to change your photo into an SVG. But your final image won’t look exactly like your photograph.
-
Requires some knowledge of code. SVG is an XML-based scene description language for graphics. Like HTML, SVG is great for people with some engineering know-how.
React and SVG
Using the <img>
tag for static SVGs
Usually it need to setup file-loader in your bundler. But It always done for you when you are using CRA or Vite.
import ReactLogo from './logo.svg';
const MyComponent = () => {
return (
<div >
<img className="MyStyle" src={ReactLogo} alt="React Logo" />
</div>
);
}
Using <svg>
element
We can use the <svg>
element by copying and pasting the contents of the .svg
file into our code. JSX supports the svg tag, allowing for the direct copy-paste of SVGs into React components without using a bundler.
const ChevronSvg = () => {
return (
<svg version="1.1" id="Layer_1"
xmlns="http://www.w3.org/2000/svg" x="0px" y="0px"
viewBox="0 0 404.257 404.257" xmlSpace="preserve">
<polygon points="386.257,114.331 202.128,252.427 18,114.331 0,138.331 202.128,289.927 404.257,138.331 "/>
<g></g>
</svg>
);
}
Using svg as component
If you use create-react-app you can use ReactComponent
:
import { ReactComponent as YourSvg } from './your-svg.svg';
const App = () => (
<div>
<YourSvg />
</div>
);
Usually you can change color like this:
.change-my-color {
fill: green;
}
const App = () => (
<div>
<YourSvg className='change-my-color'/>
</div>
);
Or using third party tool SVGR
or ReactSVG
.
Third party tools
third party tool SVGR
or ReactSVG
.
SVG Syntax
You can go to w3school to test SVG.
The ‘viewBox’ attribute
Using the viewBox
attribute to automatically create an initial user coordinate system which causes the graphic to scale to fit into the SVG viewport no matter what size the SVG viewport is.
Syntax:
viewBox = "min-x min-y width height"
Attribute Values:
- min-x: It is used to set the horizontal axis. It is used to make the SVG move on a horizontal axis (i.e Left and Right).
- min-y: It is used to set the vertical axis. It is used to make the SVG move on a vertical axis (i.e Up and Down).
- width: It is used to set the width of viewbox.
- height: It is used to set the height of viewbox.
Values of width and height: With the width and height values you can change the size of the SVG vector. If we want to change the size and make it larger, then set the value for width and height, in viewBox, smaller than the width and height properties of the SVG element.
Example
<rect>
and <circle>
descendants creating very different results in the following SVGs:
- The size of
<rect>
is defined using relative units, so the visual size of the square produced looks unchanged regardless of the viewBox value. - The radius length r attribute of the
<circle>
is the same in each case, but this user unit value is resolved against the size defined in the viewBox, producing different results in each case.
<div style='width: 100px;height: 300px;'>
<svg viewBox="0 0 100 100" xmlns="http://www.w3.org/2000/svg">
<rect x="0" y="0" width="100%" height="100%" />
<circle cx="50%" cy="50%" r="4" fill="white" />
</svg>
<svg viewBox="0 0 10 10" xmlns="http://www.w3.org/2000/svg">
<rect x="0" y="0" width="100%" height="100%" />
<circle cx="50%" cy="50%" r="4" fill="white" />
</svg>
<svg viewBox="-5 -5 10 10" xmlns="http://www.w3.org/2000/svg">
<rect x="0" y="0" width="100%" height="100%" />
<circle cx="50%" cy="50%" r="4" fill="white" />
</svg>
</div>